Exploring the Fundamentals of Time and Space Complexity: Understanding and Optimizing Algorithm Performance
Time and space complexity are crucial concepts in computer science that describe the performance of an algorithm. Understanding these concepts can help us make informed decisions about the design and implementation of our software, and can also help us choose the most appropriate algorithm for a given problem. In this blog post, we'll take a closer look at time and space complexity, and discuss some of the key points to keep in mind when analyzing the performance of an algorithm.
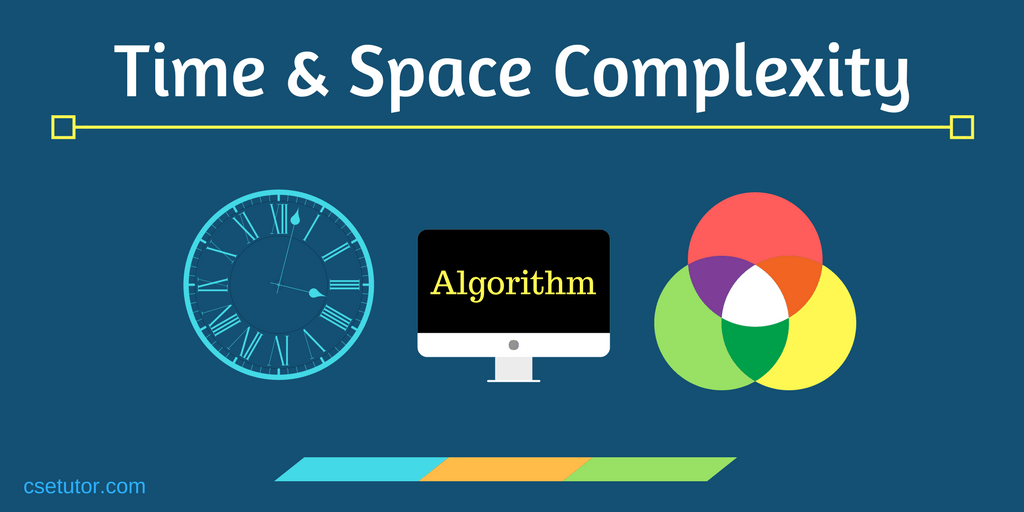
Time Complexity
Time complexity describes the amount of time it takes for an algorithm to complete, typically measured in terms of the size of the input. When analyzing the time complexity of an algorithm, we typically use big O notation, which describes the worst-case scenario.
Constant Time Complexity: An algorithm that has a constant time complexity of O(1) will take the same amount of time to execute regardless of the size of the input. For example, if you have an array and you want to access the element at index i, it will take the same amount of time regardless of the size of the array.
Linear Time Complexity: An algorithm that has a linear time complexity of O(n) will take an amount of time that increases linearly with the size of the input. For example, if you have an array and you want to iterate through all the elements, the time it takes to complete the iteration will increase linearly with the size of the array.
Logarithmic Time Complexity: An algorithm that has a logarithmic time complexity of O(log n) will take an amount of time that increases logarithmically with the size of the input. For example, if you are looking for an element in a sorted array using binary search, the time it takes to find the element will increase logarithmically with the size of the array.
Polynomial Time Complexity: An algorithm that has a polynomial time complexity of O(n^k) will take an amount of time that increases with the size of the input raised to the power of k. For example, if you are solving a problem using a brute-force approach that involves nested loops, the time it takes to solve the problem will increase with the size of the input raised to the power of the number of nested loops.
Exponential Time Complexity: An algorithm that has an exponential time complexity of O(2^n) will take an amount of time that increases exponentially with the size of the input. For example, if you are solving a problem using a recursive approach that involves a recursive call for each element of the input, the time it takes to solve the problem will increase exponentially with the size of the input.
It's important to note that the time complexity of an algorithm is not always the same as the actual running time. The actual running time will depend on the specific implementation and the specific inputs. However, the time complexity gives us a general idea of how the algorithm will perform as the size of the input increases.
Space Complexity
Space complexity describes the amount of memory an algorithm uses, typically measured in terms of the size of the input. Just like time complexity, we can use big O notation to describe the space complexity of an algorithm.
Constant Space Complexity: An algorithm that has a constant space complexity of O(1) will use the same amount of memory regardless of the size of the input. For example, if you have a variable that stores a single value, the amount of memory used will be constant regardless of the value stored.
Linear Space Complexity: An algorithm that has a linear space complexity of O(n) will use an amount of memory that increases linearly with the size of the input. For example, if you have an array and you want to store all the elements, the amount of memory used will increase linearly with the size of the array.
Logarithmic Space Complexity: An algorithm that has a logarithmic space complexity of O(log n) will use an amount of memory that increases logarithmically with the size of the input. For example, if you are using a divide-and-conquer algorithm like binary search, the amount of memory used will increase logarithmically with the size of the input.
Polynomial Space Complexity: An algorithm that has a polynomial space complexity of O(n^k) will use an amount of memory that increases with the size of the input raised to the power of k. For example, if you are using a dynamic programming approach that involves a 2-dimensional array, the amount of memory used will increase with the size of the input raised to the power of 2.
Exponential Space Complexity: An algorithm that has an exponential space complexity of O(2^n) will use an amount of memory that increases exponentially with the size of the input. For example, if you are using a recursive approach that involves a function call for each element of the input, the amount of memory used will increase exponentially with the size of the input.
Time and Space Complexity are Related
Time and space complexity are two closely related concepts in computer science. They are both used to analyze the performance of algorithms and to determine how well they will scale with increasing input size.
In general, algorithms that have a lower time complexity will also have a lower space complexity, and vice versa. This is because the time an algorithm takes to run is often directly related to the amount of memory it uses. For example, an algorithm that uses a lot of recursions will have a higher time complexity, because each recursive call takes time, and it will also have a higher space complexity because each recursive call takes up memory on the call stack.
However, there are cases where an algorithm can have a low time complexity but a high space complexity, or vice versa. For example, a sorting algorithm that uses a lot of extra memory to store temporary data may have a lower time complexity, but a higher space complexity.
In practice, when we analyze the time and space complexity of an algorithm, we often have to trade off one for the other. For example, if we need an algorithm that can handle very large inputs, we may be willing to accept a higher space complexity in order to get a lower time complexity. On the other hand, if we need an algorithm that can run on a device with limited memory, we may be willing to accept a higher time complexity in order to get a lower space complexity.
Time Complexity Vs Actual Running Time
The actual running time of an algorithm on a specific input may be different from its theoretical time complexity.
There are several factors that can affect the actual running time of an algorithm:
Input size: The actual running time of an algorithm will depend on the size of the input. An algorithm with a time complexity of O(n) will take longer to run on an input of size 100 than on an input of size 10.
Input values: The actual running time of an algorithm can also depend on the specific values of the input. For example, an algorithm that sorts an array may take less time to run on an array that is already sorted than on an array that is in reverse order.
Hardware: The actual running time of an algorithm will depend on the hardware it is running on. For example, an algorithm that runs on a faster processor will have a shorter running time than the same algorithm running on a slower processor.
Optimization: The actual running time of an algorithm can also be affected by optimization techniques such as caching, parallelization, and vectorization. These techniques can significantly improve the performance of an algorithm.
Implementation: The actual running time of an algorithm can also depend on the specific implementation. There may be multiple ways to implement the same algorithm, and the choice of the specific implementation can affect the running time.
It's important to note that big O notation is an upper bound, meaning that the actual running time of an algorithm may be faster. However, it gives us an idea of how the running time will change as the size of the input increases, and it is a useful tool for comparing the performance of different algorithms.
Importance of Analyzing Algorithms
Here are a few reasons why analyzing algorithms is important:
Optimizing performance: By analyzing algorithms, we can determine the time and space complexity of each algorithm, which can help us to identify the most efficient algorithm for a given problem. This is especially important when working with large inputs or when developing real-time systems, where the performance of the algorithm can have a significant impact on the overall system.
Understanding trade-offs: Analyzing algorithms can also help us to understand the trade-offs between different algorithms. For example, an algorithm with a lower time complexity may have a higher space complexity, or an algorithm that is easy to implement may have worse performance than a more complex algorithm.
Developing new algorithms: By analyzing existing algorithms, we can gain a deeper understanding of the underlying principles of algorithm design and use that knowledge to develop new algorithms for new problems.
Debugging: By analyzing the performance of an algorithm, we can identify any bottlenecks or inefficiencies in the algorithm and then debug it accordingly.
Scalability: By analyzing the time and space complexity of an algorithm, we can predict how well the algorithm will scale with increasing input size. This is important for building systems that can handle large inputs and changing workloads.
Conclusion
In conclusion, understanding the concepts of time and space complexity is crucial for analyzing and optimizing the performance of algorithms. Big O notation provides a theoretical measure of an algorithm's running time and space usage and allows us to compare the performance of different algorithms. However, it's important to keep in mind that the actual running time of an algorithm may be affected by various factors such as input size, input values, hardware, optimization techniques, and implementation. By understanding the trade-offs between different algorithms and using the tools provided by time and space complexity analysis, we can make informed decisions when choosing the best algorithm for a given problem. Additionally, analyzing algorithms also plays a key role in debugging, developing new algorithms, and scalability of the system. In this blog, we have explored the fundamentals of time and space complexity and discussed the importance of analyzing algorithms in order to improve performance.